NO SOBRIETY (FRA) - Here Comes The Breath (2001)
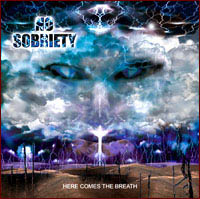
Label : Autoproduction
Sortie du Scud : 2001
Pays : France
Genre : Death / Thrash
Type : Mini CD
Playtime : 6 Titres - 27 Mins
Après quelques changements de line-up et une nouvelle approche de leur style musical qui se veut plus Death que jamais mais sans pour autant perdre leur sens de la mélodie, No Sobriety nous sert ce mini-cd avant la sortie de l'album prévu pour septembre 2002.
Un son et un mix quasi irréprochable, No Sobriety fait fort avec ce mini-cd. Riffs acérés accrocheurs, voix death caverneuse, mélodies enivrantes, combinaison basse-batterie impec... C'est sûr, No Sobriety s'affirme comme un groupe à la technicité bien présente et à la personnalité forte qui marque nos esprits de son empreinte.
En attendant l'album, lâchez vous sans modérations sur ce mini-cd.
Tout ceci est plutôt de bonne augure, ce qui prouve une fois de plus que la scène française n'est pas en reste...
Ajouté : Lundi 13 Mai 2002 Chroniqueur : Blasphy De Blasphèmar Score :     Hits: 15530
|